mirror of https://github.com/01-edu/public.git
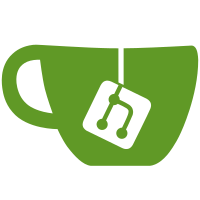
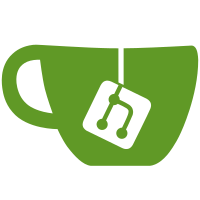
28 changed files with 144 additions and 77 deletions
@ -1,6 +1,8 @@
|
||||
use negative_spelling::*; |
||||
use dress_code::*; |
||||
|
||||
fn main() { |
||||
println!("{}", negative_spell(-1234)); |
||||
println!("{}", negative_spell(100)); |
||||
println!( |
||||
"My outfit will be: {:?}", |
||||
choose_outfit(Some(0), Ok("Dear friend, ...")) |
||||
); |
||||
} |
||||
|
@ -1,8 +1,10 @@
|
||||
use matrix_determinant::*; |
||||
|
||||
fn main() { |
||||
let matrix = [[1, 2, 4], [2, -1, 3], [4, 0, 1]]; |
||||
|
||||
println!( |
||||
"The determinant of the matrix:\n|1 2 4|\n|2 -1 3| = {}\n|4 0 1|", |
||||
matrix_determinant(matr) |
||||
matrix_determinant(matrix) |
||||
); |
||||
} |
||||
|
@ -0,0 +1,6 @@
|
||||
use negative_spelling::*; |
||||
|
||||
fn main() { |
||||
println!("{}", negative_spell(-1234)); |
||||
println!("{}", negative_spell(100)); |
||||
} |
@ -1,3 +1,5 @@
|
||||
use previousprime::*; |
||||
|
||||
fn main() { |
||||
println!("The previous prime number before 34 is: {}", prev_prime(34)); |
||||
} |
||||
|
Loading…
Reference in new issue