mirror of https://github.com/01-edu/public.git
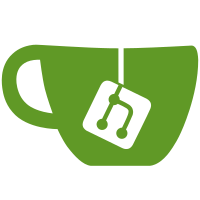
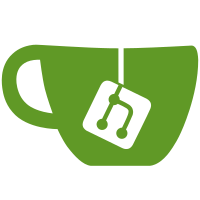
38 changed files with 534 additions and 2 deletions
@ -0,0 +1,19 @@
|
||||
use blood_types_s::{Antigen, BloodType, RhFactor}; |
||||
|
||||
fn main() { |
||||
let blood_type = BloodType { |
||||
antigen: Antigen::O, |
||||
rh_factor: RhFactor::Positive, |
||||
}; |
||||
println!("recipients of O+ {:?}", blood_type.recipients()); |
||||
println!("donors of O+ {:?}", blood_type.donors()); |
||||
let another_blood_type = BloodType { |
||||
antigen: Antigen::O, |
||||
rh_factor: RhFactor::Positive, |
||||
}; |
||||
println!( |
||||
"donors of O+ can receive from {:?} {:?}", |
||||
&another_blood_type, |
||||
blood_type.can_receive_from(&another_blood_type) |
||||
); |
||||
} |
@ -0,0 +1,31 @@
|
||||
use car_rental::*; |
||||
use std::cell::RefCell; |
||||
|
||||
fn main() { |
||||
let car_rental = RentalBusiness { |
||||
car: RefCell::new(Car { |
||||
color: "red".to_string(), |
||||
plate: "AAA".to_string(), |
||||
}), |
||||
}; |
||||
|
||||
println!("{:?}", car_rental.rent_car()); |
||||
println!("{:?}", car_rental.repair_car()); |
||||
|
||||
{ |
||||
let mut car = car_rental.repair_car(); |
||||
car.color = "blue".to_string(); |
||||
} |
||||
|
||||
println!("{:?}", car_rental.rent_car()); |
||||
|
||||
car_rental.change_car(Car { |
||||
color: "pink".to_string(), |
||||
plate: "WWW".to_string(), |
||||
}); |
||||
|
||||
println!("{:?}", car_rental.rent_car()); |
||||
|
||||
println!("{:?}", car_rental.sell_car()); |
||||
println!("{:?}", car_rental.sell_car()); |
||||
} |
@ -0,0 +1,12 @@
|
||||
use check_user_name::*; |
||||
|
||||
fn main() { |
||||
let user0 = User::new("Didier".to_string(), AccessLevel::Admin); |
||||
println!("{:?}", check_user_name(&user0)); |
||||
|
||||
let user1 = User::new("Mary".to_string(), AccessLevel::Normal); |
||||
println!("{:?}", check_user_name(&user1)); |
||||
|
||||
let user2 = User::new("John".to_string(), AccessLevel::Guest); |
||||
println!("{:?}", check_user_name(&user2)); |
||||
} |
@ -0,0 +1,10 @@
|
||||
use count_factorial_steps::count_factorial_steps; |
||||
|
||||
fn main() { |
||||
println!( |
||||
"The factorial steps of 720 = {}", |
||||
count_factorial_steps(720) |
||||
); |
||||
println!("The factorial steps of 13 = {}", count_factorial_steps(13)); |
||||
println!("The factorial steps of 6 = {}", count_factorial_steps(6)); |
||||
} |
@ -0,0 +1,9 @@
|
||||
use counting_words::counting_words; |
||||
use std::collections::HashMap; |
||||
|
||||
fn main() { |
||||
println!("{:?}", counting_words("Hello, world!")); |
||||
println!("{:?}", counting_words("“Two things are infinite: the universe and human stupidity; and I'm not sure about the universe.” |
||||
― Albert Einstein ")); |
||||
println!("{:?}", counting_words("Batman, BATMAN, batman, Stop stop")); |
||||
} |
@ -0,0 +1,29 @@
|
||||
fn main() { |
||||
let mut table = Table::new(); |
||||
println!("{}", table); |
||||
table.headers = vec![ |
||||
String::from("Model"), |
||||
String::from("Piece N°"), |
||||
String::from("In Stock"), |
||||
String::from("Description"), |
||||
]; |
||||
table.add_row(&[ |
||||
String::from("model 1"), |
||||
String::from("43-EWQE304"), |
||||
String::from("30"), |
||||
String::from("Piece for x"), |
||||
]); |
||||
table.add_row(&[ |
||||
String::from("model 2"), |
||||
String::from("98-QCVX5433"), |
||||
String::from("100000000"), |
||||
String::from("-"), |
||||
]); |
||||
table.add_row(&[ |
||||
String::from("model y"), |
||||
String::from("78-NMNH"), |
||||
String::from("60"), |
||||
String::from("nothing"), |
||||
]); |
||||
println!("{}", table); |
||||
} |
@ -0,0 +1,6 @@
|
||||
use negative_spelling::*; |
||||
|
||||
fn main() { |
||||
println!("{}", negative_spell(-1234)); |
||||
println!("{}", negative_spell(100)); |
||||
} |
@ -0,0 +1,31 @@
|
||||
use drop_the_blog::*; |
||||
use std::rc::Rc; |
||||
|
||||
fn main() { |
||||
let blog = Blog::new(); |
||||
let (id, article) = blog.new_article(String::from("Winter is coming")); |
||||
let (id1, article1) = blog.new_article(String::from("The story of the universe")); |
||||
|
||||
article.discard(); |
||||
|
||||
println!("{:?}", (blog.is_dropped(id), id, &blog.drops)); |
||||
|
||||
article1.discard(); |
||||
println!("{:?}", (blog.is_dropped(id1), id1, &blog.drops)); |
||||
|
||||
let (id2, article2) = blog.new_article(String::from("How to cook 101")); |
||||
let article2 = Rc::new(article2); |
||||
let article2_clone = article2.clone(); |
||||
|
||||
drop(article2_clone); |
||||
|
||||
println!( |
||||
"{:?}", |
||||
( |
||||
blog.is_dropped(id2), |
||||
id2, |
||||
&blog.drops, |
||||
Rc::strong_count(&article2) |
||||
) |
||||
); |
||||
} |
@ -0,0 +1,28 @@
|
||||
fn main() { |
||||
let mut table = Table::new(); |
||||
table.headers = vec![ |
||||
"Name".to_string(), |
||||
"Last Name".to_string(), |
||||
"ID Number".to_string(), |
||||
]; |
||||
table.add_row(&[ |
||||
"Adam".to_string(), |
||||
"Philips".to_string(), |
||||
"123456789".to_string(), |
||||
]); |
||||
table.add_row(&[ |
||||
"Adamaris".to_string(), |
||||
"Shelby".to_string(), |
||||
"1111123456789".to_string(), |
||||
]); |
||||
table.add_row(&[ |
||||
"Ackerley".to_string(), |
||||
"Philips".to_string(), |
||||
"123456789".to_string(), |
||||
]); |
||||
let filter_names = |col: &str| col == "Name"; |
||||
println!("{:?}", table.filter_col(filter_names)); |
||||
|
||||
let filter_philips = |lastname: &str| lastname == "Philips"; |
||||
println!("{:?}", table.filter_row("Last Name", filter_philips)); |
||||
} |
@ -0,0 +1,15 @@
|
||||
fn main() { |
||||
let mut tree = BTreeSet::new(); |
||||
tree.insert(34); |
||||
tree.insert(0); |
||||
tree.insert(9); |
||||
tree.insert(30); |
||||
println!("{:?}", flatten_tree(&tree)); |
||||
|
||||
let mut tree = BTreeSet::new(); |
||||
tree.insert("Slow"); |
||||
tree.insert("kill"); |
||||
tree.insert("will"); |
||||
tree.insert("Horses"); |
||||
println!("{:?}", flatten_tree(&tree)); |
||||
} |
@ -0,0 +1,24 @@
|
||||
use format_me::*; |
||||
|
||||
fn main() { |
||||
println!( |
||||
"{}", |
||||
Park { |
||||
name: "Les Tuileries".to_string(), |
||||
park_type: ParkType::Garden, |
||||
address: "Pl. de la Concorde".to_string(), |
||||
cap: "75001".to_string(), |
||||
state: "France".to_string() |
||||
} |
||||
); |
||||
println!( |
||||
"{}", |
||||
Park { |
||||
name: "".to_string(), |
||||
park_type: ParkType::Playground, |
||||
address: "".to_string(), |
||||
cap: "".to_string(), |
||||
state: "".to_string() |
||||
} |
||||
); |
||||
} |
@ -0,0 +1,29 @@
|
||||
use get_document_id::*; |
||||
|
||||
fn main() { |
||||
let office_ok = OfficeOne { |
||||
next_office: Ok(OfficeTwo { |
||||
next_office: Ok(OfficeThree { |
||||
next_office: Ok(OfficeFour { |
||||
document_id: Ok(13), |
||||
}), |
||||
}), |
||||
}), |
||||
}; |
||||
let office_closed = { |
||||
OfficeOne { |
||||
next_office: Ok(OfficeTwo { |
||||
next_office: Err(ErrorOffice::OfficeClose(23)), |
||||
}), |
||||
} |
||||
}; |
||||
|
||||
match office_ok.get_document_id() { |
||||
Ok(id) => println!("Found a document with id {}", id), |
||||
Err(err) => println!("Error: {:?}", err), |
||||
}; |
||||
match office_closed.get_document_id() { |
||||
Ok(id) => println!("Found a document with id {}", id), |
||||
Err(err) => println!("Error: {:?}", err), |
||||
}; |
||||
} |
@ -0,0 +1,13 @@
|
||||
fn main() { |
||||
let mut target = [5, 3, 7, 2, 1, 6, 8, 4]; |
||||
// executes the first iteration of the algorithm
|
||||
insertion_sort(&mut target, 1); |
||||
println!("{:?}", target); |
||||
|
||||
let mut target = [5, 3, 7, 2, 1, 6, 8, 4]; |
||||
let len = target.len(); |
||||
// executes len - 1 iterations of the algorithm
|
||||
// i.e. sorts the slice
|
||||
insertion_sort(&mut target, len - 1); |
||||
println!("{:?}", target); |
||||
} |
@ -0,0 +1,19 @@
|
||||
fn main() { |
||||
let a = inv_pyramid(String::from("#"), 1); |
||||
let b = inv_pyramid(String::from("a"), 2); |
||||
let c = inv_pyramid(String::from(">"), 5); |
||||
let d = inv_pyramid(String::from("&"), 8); |
||||
|
||||
for v in a.iter() { |
||||
println!("{:?}", v); |
||||
} |
||||
for v in b.iter() { |
||||
println!("{:?}", v); |
||||
} |
||||
for v in c.iter() { |
||||
println!("{:?}", v); |
||||
} |
||||
for v in d.iter() { |
||||
println!("{:?}", v); |
||||
} |
||||
} |
@ -0,0 +1,24 @@
|
||||
use lucas_number::lucas_number; |
||||
|
||||
fn main() { |
||||
println!( |
||||
"The element in the position {} in Lucas Numbres is {}", |
||||
2, |
||||
lucas_number(2) |
||||
); |
||||
println!( |
||||
"The element in the position {} in Lucas Numbres is {}", |
||||
5, |
||||
lucas_number(5) |
||||
); |
||||
println!( |
||||
"The element in the position {} in Lucas Numbres is {}", |
||||
10, |
||||
lucas_number(10) |
||||
); |
||||
println!( |
||||
"The element in the position {} in Lucas Numbres is {}", |
||||
13, |
||||
lucas_number(13) |
||||
); |
||||
} |
@ -0,0 +1,17 @@
|
||||
fn main() { |
||||
let mut list = Queue::new(); |
||||
list.add(String::from("Marie"), 20); |
||||
list.add(String::from("Monica"), 15); |
||||
list.add(String::from("Ana"), 5); |
||||
list.add(String::from("Alice"), 35); |
||||
println!("{:?}", list); |
||||
|
||||
println!("{:?}", list.search("Marie")); |
||||
println!("{:?}", list.search("Alice")); |
||||
println!("{:?}", list.search("someone")); |
||||
|
||||
println!("removed {:?}", list.rm()); |
||||
println!("list {:?}", list); |
||||
list.invert_queue(); |
||||
println!("invert {:?}", list); |
||||
} |
@ -0,0 +1,8 @@
|
||||
fn main() { |
||||
let matrix = [[1, 2, 4], [2, -1, 3], [4, 0, 1]]; |
||||
|
||||
println!( |
||||
"The determinant of the matrix:\n|1 2 4|\n|2 -1 3| = {}\n|4 0 1|", |
||||
matrix_determinant(matr) |
||||
); |
||||
} |
@ -0,0 +1,4 @@
|
||||
fn main() { |
||||
let matrix = Matrix::new(&[&[1, 2, 3], &[4, 5, 6], &[7, 8, 9]]); |
||||
println!("{}", matrix); |
||||
} |
@ -0,0 +1,7 @@
|
||||
use matrix_multiplication::*; |
||||
|
||||
fn main() { |
||||
let matrix = Matrix((1, 3), (4, 5)); |
||||
println!("Original matrix {:?}", matrix); |
||||
println!("Matrix after multiply {:?}", multiply(matrix, 3)); |
||||
} |
@ -0,0 +1,5 @@
|
||||
use min_and_max::min_and_max; |
||||
|
||||
fn main() { |
||||
println!("Minimum and maximum are: {:?}", min_and_max(9, 2, 4)); |
||||
} |
@ -0,0 +1,7 @@
|
||||
use modify_letter::*modify_letter*; |
||||
|
||||
fn main() { |
||||
println!("{}", remove_letter_sensitive("Jojhn jis sljeepjjing", 'j')); |
||||
println!("{}", remove_letter_insensitive("JaimA ais swiaAmmingA", 'A')); |
||||
println!("{}", swap_letter_case("byE bye", 'e')); |
||||
} |
@ -0,0 +1,14 @@
|
||||
use moving_targets::*; |
||||
|
||||
fn main() { |
||||
let mut field = Field::new(); |
||||
|
||||
println!("{:?}", field.pop()); |
||||
field.push(Target { size: 12, xp: 2 }); |
||||
println!("{:?}", *field.peek().unwrap()); |
||||
field.push(Target { size: 24, xp: 4 }); |
||||
println!("{:?}", field.pop()); |
||||
let last_target = field.peek_mut().unwrap(); |
||||
*last_target = Target { size: 2, xp: 0 }; |
||||
println!("{:?}", field.pop()); |
||||
} |
@ -0,0 +1,4 @@
|
||||
fn main() { |
||||
println!("The next prime after 4 is: {}", next_prime(4)); |
||||
println!("The next prime after 11 is: {}", next_prime(11)); |
||||
} |
@ -0,0 +1,9 @@
|
||||
use office_worker::*; |
||||
|
||||
fn main() { |
||||
println!("New worker: {:?}", OfficeWorker::from("Manuel,23,admin")); |
||||
println!( |
||||
"New worker: {:?}", |
||||
OfficeWorker::from("Jean Jacques,44,guest") |
||||
); |
||||
} |
@ -0,0 +1,39 @@
|
||||
pub use library::books::Book; |
||||
pub use library::writers::Writer; |
||||
|
||||
fn main() { |
||||
let mut writer_a = Writer { |
||||
first_name: "William".to_string(), |
||||
last_name: "Shakespeare".to_string(), |
||||
books: vec![ |
||||
Book { |
||||
title: "Hamlet".to_string(), |
||||
year: 1600, |
||||
}, |
||||
Book { |
||||
title: "Othelo".to_string(), |
||||
year: 1603, |
||||
}, |
||||
Book { |
||||
title: "Romeo and Juliet".to_string(), |
||||
year: 1593, |
||||
}, |
||||
Book { |
||||
title: "MacBeth".to_string(), |
||||
year: 1605, |
||||
}, |
||||
], |
||||
}; |
||||
|
||||
println!("Before ordering"); |
||||
for b in &writer_a.books { |
||||
println!("{:?}", b.title); |
||||
} |
||||
|
||||
order_books(&mut writer_a); |
||||
|
||||
println!("\nAfter ordering"); |
||||
for b in writer_a.books { |
||||
println!("{:?}", b.title); |
||||
} |
||||
} |
@ -0,0 +1,14 @@
|
||||
use organize_garage::*; |
||||
|
||||
fn main() { |
||||
let mut garage = Garage { |
||||
left: Some(5), |
||||
right: Some(2), |
||||
}; |
||||
|
||||
println!("{:?}", garage); |
||||
garage.move_to_right(); |
||||
println!("{:?}", garage); |
||||
garage.move_to_left(); |
||||
println!("{:?}", garage); |
||||
} |
@ -0,0 +1,18 @@
|
||||
use own_and_return::*; |
||||
|
||||
pub struct Film { |
||||
pub name: String, |
||||
} |
||||
|
||||
fn main() { |
||||
let my_film = Film { |
||||
name: "Terminator".toString(), |
||||
}; |
||||
println!("{}", take_film_name(/* to be implemented */)); |
||||
// the order of the print statements is intentional, if your implementation is correct,
|
||||
// you should have a compile error because my_film was consumed
|
||||
println!("{}", read_film_name(/* to be implemented */)); |
||||
println!("{}", take_film_name(/*to be implemented*/)) |
||||
// you can test this function by commenting out the first print statement,
|
||||
// you should see the expected output without errors in this case
|
||||
} |
@ -0,0 +1,6 @@
|
||||
fn main() { |
||||
println!( |
||||
"Partial sums of [5, 18, 3, 23] is : {:?}", |
||||
parts_sums(&[5, 18, 3, 23]) |
||||
); |
||||
} |
@ -0,0 +1,3 @@
|
||||
fn main() { |
||||
println!("The previous prime number before 34 is: {}", prev_prime(34)); |
||||
} |
@ -0,0 +1,7 @@
|
||||
use prime_checker::*; |
||||
|
||||
fn main() { |
||||
println!("Is {} prime? {:?}", 2, prime_checker(2)); |
||||
println!("Is {} prime? {:?}", 14, prime_checker(14)); |
||||
println!("Is {} prime? {:?}", 2147483647, prime_checker(2147483647)); |
||||
} |
@ -0,0 +1,15 @@
|
||||
use profanity_filter::*; |
||||
|
||||
fn main() { |
||||
let m0 = Message::new("hello there".to_string(), "toby".to_string()); |
||||
println!("{:?}", check_ms(&m0)); |
||||
|
||||
let m1 = Message::new("".to_string(), "toby".to_string()); |
||||
println!("{:?}", check_ms(&m1)); |
||||
|
||||
let m2 = Message::new("you are stupid".to_string(), "toby".to_string()); |
||||
println!("{:?}", check_ms(&m2)); |
||||
|
||||
let m3 = Message::new("stupid".to_string(), "toby".to_string()); |
||||
println!("{:?}", check_ms(&m3)); |
||||
} |
@ -0,0 +1,17 @@
|
||||
fn main() { |
||||
let white_queen = Queen::new(ChessPosition::new(2, 2).unwrap()); |
||||
let black_queen = Queen::new(ChessPosition::new(0, 4).unwrap()); |
||||
|
||||
println!( |
||||
"Is it possible for the queens to attack each other? => {}", |
||||
white_queen.can_attack(&black_queen) |
||||
); |
||||
|
||||
let white_queen = Queen::new(ChessPosition::new(1, 2).unwrap()); |
||||
let black_queen = Queen::new(ChessPosition::new(0, 4).unwrap()); |
||||
|
||||
println!( |
||||
"Is it possible for the queens to attack each other? => {}", |
||||
white_queen.can_attack(&black_queen) |
||||
); |
||||
} |
@ -0,0 +1,4 @@
|
||||
fn main() { |
||||
println!("{}", reverse_it(123)); |
||||
println!("{}", reverse_it(-123)); |
||||
} |
@ -0,0 +1,9 @@
|
||||
use rot21::rot21; |
||||
|
||||
fn main() { |
||||
println!("The letter \"a\" becomes: {}", rot21("a")); |
||||
println!("The letter \"m\" becomes: {}", rot21("m")); |
||||
println!("The word \"MISS\" becomes: {}", rot21("MISS")); |
||||
println!("Your cypher wil be: {}", rot21("Testing numbers 1 2 3")); |
||||
println!("Your cypher wil be: {}", rot21("rot21 works!")); |
||||
} |
@ -0,0 +1,11 @@
|
||||
fn main() { |
||||
println!( |
||||
"\"sec yCtoadle\" size=2 -> {:?}", |
||||
scytale_decoder("sec yCtoadle".to_string(), 2) |
||||
); |
||||
|
||||
println!( |
||||
"\"steoca dylCe\" size=4 -> {:?}", |
||||
scytale_decoder("steoca dylCe".to_string(), 4) |
||||
); |
||||
} |
@ -0,0 +1,15 @@
|
||||
use smallest::smallest; |
||||
use std::collections::HashMap; |
||||
|
||||
fn main() { |
||||
let mut hash = HashMap::new(); |
||||
hash.insert("Cat", 122); |
||||
hash.insert("Dog", 333); |
||||
hash.insert("Elephant", 334); |
||||
hash.insert("Gorilla", 14); |
||||
|
||||
println!( |
||||
"The smallest of the elements in the HashMap is {}", |
||||
smallest(hash) |
||||
); |
||||
} |
Loading…
Reference in new issue