mirror of https://github.com/01-edu/public.git
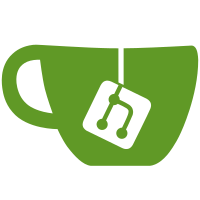
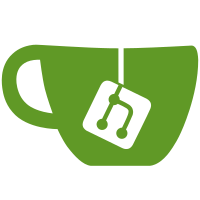
40 changed files with 1848 additions and 0 deletions
@ -0,0 +1,46 @@
|
||||
# Boolean |
||||
|
||||
## Instructions |
||||
|
||||
Create a `.go` file and copy the code below into our file |
||||
|
||||
- The main task is to return a working program. |
||||
|
||||
```go |
||||
|
||||
func printStr(str string) { |
||||
arrayStr := []rune(str) |
||||
|
||||
for i := 0; i < len(arrayStr); i++ { |
||||
z01.PrintRune(arrayStr[i]) |
||||
} |
||||
z01.PrintRune('\n') |
||||
} |
||||
|
||||
func isEven(nbr int) boolean { |
||||
|
||||
if even(nbr) == 1 { |
||||
return yes |
||||
} else { |
||||
return no |
||||
} |
||||
} |
||||
|
||||
func main() { |
||||
|
||||
if isEven(lengthOfArg) == 1 { |
||||
printStr(EvenMsg) |
||||
} else { |
||||
printStr(OddMsg) |
||||
} |
||||
} |
||||
``` |
||||
|
||||
## Expected output |
||||
```go |
||||
I have an even number of arguments |
||||
``` |
||||
## Or |
||||
```go |
||||
I have an odd number of arguments |
||||
``` |
@ -0,0 +1,37 @@
|
||||
# Cat |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that does the same thing as the system's `cat` command-line. |
||||
|
||||
- You don't have to handle options. |
||||
|
||||
- But if just call the program with out arguments it should take a input and print it back |
||||
|
||||
- In the program folder create two files named `quest8.txt` and `quest8T.txt`. |
||||
|
||||
- Copy to the `quest8.txt` file this : |
||||
|
||||
- "Programming is a skill best acquired by pratice and example rather than from books" by Alan Turing |
||||
|
||||
- Copy to the `quest8T.txt` file this : |
||||
|
||||
- "Alan Mathison Turing was an English mathematician, computer scientist, logician, cryptanalyst. Turing was highly influential in the development of theoretical computer science, providing a formalisation of the concepts of algorithm and computation with the Turing machine, which can be considered a model of a general-purpose computer. Turing is widely considered to be the father of theoretical computer science and artificial intelligence." |
||||
|
||||
- In case of error it should print the error. |
||||
|
||||
## Output: |
||||
|
||||
```console |
||||
student@ubuntu:~/student/test$ go build |
||||
student@ubuntu:~/student/test$ ./test |
||||
Hello |
||||
Hello |
||||
student@ubuntu:~/student/test$ ./test quest8.txt |
||||
"Programming is a skill best acquired by pratice and example rather than from books" by Alan Turing |
||||
student@ubuntu:~/student/test$ ./test quest8.txt quest8T.txt |
||||
"Programming is a skill best acquired by pratice and example rather than from books" by Alan Turing |
||||
|
||||
"Alan Mathison Turing was an English mathematician, computer scientist, logician, cryptanalyst. Turing was highly influential in the development of theoretical computer science, providing a formalisation of the concepts of algorithm and computation with the Turing machine, which can be considered a model of a general-purpose computer. Turing is widely considered to be the father of theoretical computer science and artificial intelligence." |
||||
|
||||
``` |
@ -0,0 +1,46 @@
|
||||
# ROT 14 |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `rot14` that returns the string within the parameter but transformed into a rot14 string. |
||||
|
||||
- If you not certain what we are talking about, there is a rot13 already. |
||||
|
||||
## Expected function |
||||
|
||||
```go |
||||
func rot14(str string) string { |
||||
|
||||
} |
||||
``` |
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func main() { |
||||
result := rot14("Hello How are You") |
||||
arrayRune := []rune(result) |
||||
|
||||
for _, s := range arrayRune { |
||||
z01.PrintRune(s) |
||||
} |
||||
z01.PrintRune('\n') |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
Vszzc Vck ofs Mci |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,41 @@
|
||||
# Compact |
||||
|
||||
## Instructions |
||||
|
||||
Write a function that will take a pointer to a array as parameter and overwrites any element that points to `nil`. |
||||
|
||||
- If you not sure what the function does. It exists in Ruby. |
||||
|
||||
## Expected functions |
||||
|
||||
```go |
||||
func Compact(ptr *[]string, length int) int { |
||||
|
||||
} |
||||
``` |
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import fmt |
||||
|
||||
func main() { |
||||
array := []string{"hello", " ", "there", " ", "bye"} |
||||
|
||||
ptr := &array |
||||
fmt.Println(Compact(ptr, len(array))) |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
3 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,17 @@
|
||||
# countdown |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that displays all digits in descending order, followed by a |
||||
newline. |
||||
|
||||
## Expected main and function for the program |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
9876543210 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,46 @@
|
||||
# createelem |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `CreateElem` that creates a new element of type`Node`. |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
} |
||||
|
||||
func CreateElem(n *Node, value int) { |
||||
|
||||
} |
||||
|
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
n := &node{} |
||||
n.CreateElem(1234) |
||||
fmt.Println(n) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
&{1234} |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,17 @@
|
||||
# dispfirstpar |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that takes strings as arguments, and displays its first argument. |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test hello there |
||||
hello |
||||
student@ubuntu:~/piscine/test$ ./test "hello there" how are you |
||||
hello there |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,19 @@
|
||||
# displastpar |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that takes strings as arguments, and displays its last argument. |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test hello there |
||||
there |
||||
student@ubuntu:~/piscine/test$ ./test "hello there" how are you |
||||
you |
||||
student@ubuntu:~/piscine/test$ ./test "hello there" |
||||
hello there |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,22 @@
|
||||
# displaya |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that takes a string, and displays the first 'a' character it |
||||
encounters in it, followed by a newline. If there are no 'a' characters in the |
||||
string, the program just writes a newline. If the number of parameters is not |
||||
1, the program displays 'a' followed by a newline. |
||||
|
||||
## Expected main and function for the program |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "abc" |
||||
a |
||||
student@ubuntu:~/piscine/test$ ./test "bcvbvA" |
||||
a |
||||
student@ubuntu:~/piscine/test$ ./test "nbv" |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,25 @@
|
||||
# Display File |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that displays, on the standard output, only the content of the file given as argument. |
||||
|
||||
- Create a file `quest8.txt` and write into the file `Almost there!!` |
||||
|
||||
- The argument of the program should be the name of the file, in this case, `quest8.txt`. |
||||
|
||||
- In case of error it should print: |
||||
- `File name missing`. |
||||
- `Too many arguments`. |
||||
|
||||
## Output: |
||||
|
||||
```console |
||||
student@ubuntu:~/student/test$ go build |
||||
student@ubuntu:~/student/test$ ./test |
||||
File name missing |
||||
student@ubuntu:~/student/test$ ./test quest8.txt main.go |
||||
Too many arguments |
||||
student@ubuntu:~/student/test$ ./test quest8.txt |
||||
Almost there!! |
||||
``` |
@ -0,0 +1,22 @@
|
||||
# displayz |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that takes a string, and displays the first 'a' character it |
||||
encounters in it, followed by a newline. If there are no 'a' characters in the |
||||
string, the program just writes a newline. If the number of parameters is not |
||||
1, the program displays 'a' followed by a newline. |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "abc" |
||||
z |
||||
student@ubuntu:~/piscine/test$ ./test "bcvbvZ" |
||||
z |
||||
student@ubuntu:~/piscine/test$ ./test "nbz" |
||||
z |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
z |
||||
``` |
@ -0,0 +1,26 @@
|
||||
# firstword |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that takes a string and displays its first word, followed by a newline. |
||||
|
||||
- A word is a section of string delimited by spaces or by the start/end of the string. |
||||
|
||||
- The output will be followed by a newline. |
||||
|
||||
- If the number of parameters is not 1, or if there are no words, simply display a newline. |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "hello there" |
||||
hello |
||||
student@ubuntu:~/piscine/test$ ./test "hello ......... bye" |
||||
hello |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
|
||||
student@ubuntu:~/piscine/test$ ./test "hello" "there" |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,65 @@
|
||||
# Fix the Main |
||||
|
||||
## Instructions |
||||
|
||||
Write and fix the folloing functions. |
||||
|
||||
## Expected functions |
||||
|
||||
```go |
||||
func PutStr(str string) { |
||||
arrayRune := []rune(str) |
||||
for _, s := range arrayRune { |
||||
z01.PrintRune(s) |
||||
} |
||||
} |
||||
|
||||
func CloseDoor(ptrDoor *Door) bool { |
||||
PutStr("Door closing...") |
||||
state = CLOSE |
||||
return true |
||||
} |
||||
|
||||
func IsDoorOpen(Door Door) { |
||||
PutStr("Door is open ?") |
||||
return Door.state = OPEN |
||||
} |
||||
|
||||
func IsDoorClose(ptrDoor *Door) bool { |
||||
PutStr("Door is close ?") |
||||
} |
||||
``` |
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
func main() { |
||||
door := &Door{} |
||||
|
||||
OpenDoor(door) |
||||
if IsDoorClose(door) { |
||||
OpenDoor(door) |
||||
} |
||||
if IsDoorOpen(door) { |
||||
CloseDoor(door) |
||||
} |
||||
if door.state == OPEN { |
||||
CloseDoor(door) |
||||
} |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
Door Opening... |
||||
Door is close ? |
||||
Door is open ? |
||||
Door closing... |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,16 @@
|
||||
# hello |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that displays "Hello World!". |
||||
|
||||
## Expected main and function for the program |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
Hello World! |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,21 @@
|
||||
# switchcase |
||||
|
||||
## Instructions |
||||
|
||||
|
||||
Write a program that takes two strings and displays, without doubles, the characters that appear in both strings, in the order they appear in the first one. |
||||
|
||||
- The display will be followed by a `\n`. |
||||
|
||||
- If the number of arguments is not 2, the program displays `\n`. |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "padinton" "paqefwtdjetyiytjneytjoeyjnejeyj" |
||||
padinto |
||||
student@ubuntu:~/piscine/test$ ./test ddf6vewg64f twthgdwthdwfteewhrtag6h4ffdhsd |
||||
df6ewg4 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,61 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListAt` that haves one pointer to the list, `l`, and an `int` as parameters. This function should print a `Node` of the linked list, depending on the number, `nbr`. |
||||
|
||||
- In case of error it should print `nil` |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
Next *Node |
||||
} |
||||
|
||||
|
||||
func ListAt(l *Node, nbr int) *Node{ |
||||
|
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
link := &Node{} |
||||
|
||||
ListPushBack(link, "hello") |
||||
ListPushBack(link, "how are") |
||||
ListPushBack(link, "you") |
||||
ListPushBack(link, 1) |
||||
|
||||
fmt.Println() |
||||
|
||||
fmt.Println(ListAt(link, 3).Data) |
||||
fmt.Println(ListAt(link, 1).Data) |
||||
fmt.Println(ListAt(link, 7)) |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
you |
||||
hello |
||||
<nil> |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,78 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListClear` that delets all `nodes` from a linked list, deleting the link between the list. |
||||
|
||||
- Tip: assign the list's pointer to nil |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
Next *Node |
||||
} |
||||
|
||||
type List struct { |
||||
Head *Node |
||||
Tail *Node |
||||
} |
||||
|
||||
func ListClear(l *List) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
type List = piscine.List |
||||
type Node = piscine.Node |
||||
|
||||
func PrintList(l *List) { |
||||
link := l.Head |
||||
for link != nil { |
||||
fmt.Print(link.Data, " -> ") |
||||
link = link.Next |
||||
} |
||||
fmt.Println(nil) |
||||
} |
||||
|
||||
func main() { |
||||
link := &List{} |
||||
|
||||
piscine.ListPushBack(link, "I") |
||||
piscine.ListPushBack(link, 1) |
||||
piscine.ListPushBack(link, "something") |
||||
piscine.ListPushBack(link, 2) |
||||
|
||||
fmt.Println("------list------") |
||||
PrintList(link) |
||||
piscine.ListClear(link) |
||||
fmt.Println("------updated list------") |
||||
PrintList(link) |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
------list------ |
||||
I -> 1 -> something -> 2 -> <nil> |
||||
------updated list------ |
||||
<nil> |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,64 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListFind` that returns the value of the first link that the function in the arguments its equal. |
||||
|
||||
- For this you shoud use the function `CompStr`. |
||||
|
||||
- Use pointers when ever you can. |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
Next *Node |
||||
} |
||||
|
||||
type List struct { |
||||
Head *Node |
||||
Tail *Node |
||||
} |
||||
|
||||
func CompStr(l *list) bool { |
||||
|
||||
} |
||||
|
||||
func ListFind(l *List, comp func(l *List) bool) *interface{} { |
||||
|
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
link := &List{} |
||||
|
||||
piscine.ListPushBack(link, 1) |
||||
piscine.ListPushBack(link, "hello") |
||||
piscine.ListPushBack(link, "hello2") |
||||
piscine.ListPushBack(link, "hello3") |
||||
|
||||
fmt.Println(piscine.ListFind(link, CompStr)) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
hello |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,65 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListForEach` that applies a function given as argument to the information within each of the list's links. |
||||
|
||||
- The function given as argument must have a pointer as argument: `l *list` |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
Next *Node |
||||
} |
||||
|
||||
type List struct { |
||||
Head *Node |
||||
Tail *Node |
||||
} |
||||
|
||||
func ListForEach(l *list, f func(l *list)) { |
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
link := &List{} |
||||
|
||||
piscine.ListPushBack(link, 1) |
||||
piscine.ListPushBack(link, 2) |
||||
piscine.ListPushBack(link, 3) |
||||
piscine.ListPushBack(link, 4) |
||||
|
||||
piscine.ListForEach(link, piscine.ListReverse) |
||||
|
||||
for link.Head != nil { |
||||
fmt.Println(link.Head.Data) |
||||
link.Head = link.Head.Next |
||||
} |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
4 |
||||
3 |
||||
2 |
||||
1 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,104 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListForEachIf` that applies a function given as argument to the information within some links of the list. |
||||
|
||||
- For this you will have to create a function `CompStr`, that returns a `bool`, to compare each elemente of the linked list, to see if it is a string, and than apply the function in the argument of `ListForEachIf`. |
||||
|
||||
- The function given as argument as to have a pointer as argument: `l *list`. |
||||
|
||||
- Use pointers wen ever you can. |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type node struct { |
||||
data interface{} |
||||
next *node |
||||
} |
||||
|
||||
type list struct { |
||||
head *node |
||||
tail *node |
||||
} |
||||
|
||||
func CompStr(l *list) bool { |
||||
|
||||
} |
||||
|
||||
func ListForEachIf(l *list, f func(l *list), comp func(l *list) bool) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func PrintElem(l *list) { |
||||
fmt.Println(l.head.data) |
||||
} |
||||
|
||||
func StringToInt(l *list) { |
||||
count := 1 |
||||
l.head.data = count |
||||
} |
||||
|
||||
func PrintList(l *list) { |
||||
m := l.head |
||||
for m != nil { |
||||
fmt.Print(m.data, " -> ") |
||||
m = m.next |
||||
} |
||||
|
||||
fmt.Print(l.tail) |
||||
} |
||||
func main() { |
||||
link := &list{} |
||||
|
||||
piscine.ListPushBack(link, 1) |
||||
piscine.ListPushBack(link, "hello") |
||||
piscine.ListPushBack(link, 3) |
||||
piscine.ListPushBack(link, "there") |
||||
piscine.ListPushBack(link, 23) |
||||
piscine.ListPushBack(link, "!") |
||||
piscine.ListPushBack(link, 54) |
||||
|
||||
PrintAllList(link) |
||||
|
||||
fmt.Println() |
||||
fmt.Println("--------function applied--------") |
||||
piscine.ListForEachIf(link, PrintElem, CompStr) |
||||
|
||||
piscine.ListForEachIf(link, StringToInt, CompStr) |
||||
|
||||
fmt.Println("--------function applied--------") |
||||
PrintAllList(link) |
||||
|
||||
fmt.Println() |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
1 -> hello -> 3 -> there -> 23 -> ! -> 54 -> <nil> |
||||
--------function applied-------- |
||||
hello |
||||
there |
||||
! |
||||
--------function applied-------- |
||||
1 -> 1 -> 3 -> 1 -> 23 -> 1 -> 54 -> <nil> |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,59 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListLast` that returns the last element of the linked list. |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
Next *Node |
||||
} |
||||
|
||||
type List struct { |
||||
Head *Node |
||||
Tail *Node |
||||
} |
||||
|
||||
func ListLast(l *list) *list { |
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
|
||||
func main() { |
||||
link := &list{} |
||||
link2 := &list{} |
||||
|
||||
piscine.ListPushBack(link, "three") |
||||
piscine.ListPushBack(link, 3) |
||||
piscine.ListPushBack(link, "1") |
||||
|
||||
fmt.Println(piscine.ListLast(link).head) |
||||
fmt.Println(piscine.ListLast(link2).head) |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
&{1 <nil>} |
||||
<nil> |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,78 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListMerge` that places elements of a list `l2` at the end of an other list `l1`. |
||||
|
||||
- You can't create new elements! |
||||
|
||||
- Use pointers when ever you can. |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type NodeL struct { |
||||
Data interface{} |
||||
Next *NodeL |
||||
} |
||||
|
||||
type List struct { |
||||
Head *NodeL |
||||
Tail *NodeL |
||||
} |
||||
|
||||
func listMerge(l1 *List, l2 *List) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
func PrintList(l *List) { |
||||
m := l.Head |
||||
for m != nil { |
||||
fmt.Print(m.Data, " -> ") |
||||
m = m.Next |
||||
} |
||||
|
||||
fmt.Print(nil) |
||||
fmt.Println() |
||||
} |
||||
|
||||
func main() { |
||||
link := &List{} |
||||
link2 := &List{} |
||||
|
||||
student.ListPushBack(link, "a") |
||||
student.ListPushBack(link, "b") |
||||
student.ListPushBack(link, "c") |
||||
student.ListPushBack(link, "d") |
||||
|
||||
student.ListPushBack(link2, "e") |
||||
student.ListPushBack(link2, "f") |
||||
student.ListPushBack(link2, "g") |
||||
student.ListPushBack(link2, "h") |
||||
|
||||
student.ListMerge(link, link2) |
||||
PrintList(link) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/test$ go build |
||||
student@ubuntu:~/student/test$ ./test |
||||
a -> b -> c -> d -> e -> f -> g -> h -> <nil> |
||||
student@ubuntu:~/student/test$ |
||||
``` |
@ -0,0 +1,60 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListPushBack` that inserts a new element `Node` at the end of the list, using the structure `List` |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
Next *Node |
||||
} |
||||
|
||||
type List struct { |
||||
Head *Node |
||||
Tail *Node |
||||
} |
||||
|
||||
func ListPushBack(l *List, data interface{}) { |
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
|
||||
link := &List{} |
||||
|
||||
piscine.ListPushBack(link, "Hello") |
||||
piscine.ListPushBack(link, "man") |
||||
piscine.ListPushBack(link, "how are you") |
||||
|
||||
for link.Head != nil { |
||||
fmt.Println(link.Head.Data) |
||||
link.Head = link.Head.Next |
||||
} |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
Hello |
||||
man |
||||
how are you |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,60 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListPushBack` that inserts a new element `node` at the beginning of the list using `list` |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
Next *Node |
||||
} |
||||
|
||||
type List struct { |
||||
Head *Node |
||||
Tail *Node |
||||
} |
||||
|
||||
func ListPushFront(l *list, data interface{}) { |
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
|
||||
link := &list{} |
||||
|
||||
piscine.ListPushFront(link, "Hello") |
||||
piscine.ListPushFront(link, "man") |
||||
piscine.ListPushFront(link, "how are you") |
||||
|
||||
for link.head != nil { |
||||
fmt.Println(link.head.data) |
||||
link.head = link.head.next |
||||
} |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
how are you |
||||
man |
||||
Hello |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,22 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that creates a new linked list and includes each command-line argument in to the list. |
||||
|
||||
- The first argument should be at the end of the list |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./listpushparams choumi is the best cat |
||||
cat |
||||
best |
||||
the |
||||
is |
||||
choumi |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,101 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListRemoveIf` that removes all elements that are equal to the `data_ref` introduced in the argument of the function. |
||||
|
||||
- Use pointers wen ever you can. |
||||
|
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type NodeL struct { |
||||
Data interface{} |
||||
Next *NodeL |
||||
} |
||||
|
||||
type List struct { |
||||
Head *NodeL |
||||
Tail *NodeL |
||||
} |
||||
|
||||
func ListPushFront(l *List, data interface{}) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func PrintList(l *List) { |
||||
m := l.Head |
||||
for m != nil { |
||||
fmt.Print(m.Data, " -> ") |
||||
m = m.Next |
||||
} |
||||
|
||||
fmt.Print(nil) |
||||
fmt.Println() |
||||
} |
||||
|
||||
func main() { |
||||
link := &List{} |
||||
link2 := &List{} |
||||
link3 := &List{} |
||||
|
||||
|
||||
fmt.Println("----normal state----") |
||||
student.ListPushBack(link2, 1) |
||||
PrintList(link2) |
||||
ListRemoveIf(link2, 1) |
||||
fmt.Println("------answer-----") |
||||
PrintList(link) |
||||
fmt.Println() |
||||
|
||||
fmt.Println("----normal state----") |
||||
student.ListPushBack(link, 1) |
||||
student.ListPushBack(link, "Hello") |
||||
student.ListPushBack(link, 1) |
||||
student.ListPushBack(link, "There") |
||||
student.ListPushBack(link, 1) |
||||
student.ListPushBack(link, 1) |
||||
student.ListPushBack(link, "How") |
||||
student.ListPushBack(link, 1) |
||||
student.ListPushBack(link, "are") |
||||
student.ListPushBack(link, "you") |
||||
student.ListPushBack(link, 1) |
||||
PrintList(link) |
||||
|
||||
ListRemoveIf(link, 1) |
||||
fmt.Println("------answer-----") |
||||
PrintList(link) |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/test$ go build |
||||
student@ubuntu:~/student/test$ ./test |
||||
----normal state---- |
||||
1 -> <nil> |
||||
------answer----- |
||||
<nil> |
||||
|
||||
----normal state---- |
||||
1 -> Hello -> 1 -> There -> 1 -> 1 -> How -> 1 -> are -> you -> 1 -> <nil> |
||||
------answer----- |
||||
Hello -> There -> How -> are -> you -> <nil> |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,65 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListReverse` that reverses the elements order of a given linked list. |
||||
|
||||
- Use pointers when ever you can |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
Next *Node |
||||
} |
||||
|
||||
type List struct { |
||||
Head *Node |
||||
Tail *Node |
||||
} |
||||
|
||||
func ListReverse(l *list) { |
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
link := &List{} |
||||
|
||||
listPushBack(link, 1) |
||||
listPushBack(link, 2) |
||||
listPushBack(link, 3) |
||||
listPushBack(link, 4) |
||||
|
||||
listReverse(link) |
||||
|
||||
for link.Head != nil { |
||||
fmt.Println(link.Head.Data) |
||||
link.Head = link.Head.Next |
||||
} |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
4 |
||||
3 |
||||
2 |
||||
1 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,57 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListSize` that returns the number of elements in the list. |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Node struct { |
||||
Data interface{} |
||||
Next *Node |
||||
} |
||||
|
||||
type List struct { |
||||
Head *Node |
||||
Tail *Node |
||||
} |
||||
|
||||
func ListSize(l *List) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func main() { |
||||
link := &List{} |
||||
|
||||
piscine.ListPushFront(link, "Hello") |
||||
piscine.ListPushFront(link, "2") |
||||
piscine.ListPushFront(link, "you") |
||||
piscine.ListPushFront(link, "man") |
||||
|
||||
fmt.Println(piscine.ListSize(link)) |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
4 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,91 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `ListSort` that sorts the linked list by ascending order. |
||||
|
||||
- This time you only will have the `Nodee` structure. |
||||
|
||||
- Try to use recursive. |
||||
|
||||
- Use pointers when ever you can. |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Nodee struct { |
||||
Data int |
||||
Next *Nodee |
||||
} |
||||
|
||||
func ListSort(l *NodeL) *NodeL { |
||||
|
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
student ".." |
||||
) |
||||
|
||||
//Prints the list |
||||
func PrintList(l *Nodee) { |
||||
m := l |
||||
for m != nil { |
||||
fmt.Print(m.Data, " -> ") |
||||
m = m.next |
||||
} |
||||
|
||||
fmt.Print(nil) |
||||
fmt.Println() |
||||
} |
||||
|
||||
//insert elements |
||||
func listPushBack(l *Nodee, Data int) { |
||||
|
||||
n := &Nodee{} |
||||
n.Data = Data |
||||
n.next = nil |
||||
|
||||
if l == nil { |
||||
l = n |
||||
return |
||||
} |
||||
|
||||
iterator := l |
||||
for iterator.next != nil { |
||||
iterator = iterator.next |
||||
} |
||||
iterator.next = n |
||||
} |
||||
|
||||
func main() { |
||||
link := &Nodee{} |
||||
|
||||
listPushBack(link, 5) |
||||
listPushBack(link, 4) |
||||
listPushBack(link, 3) |
||||
listPushBack(link, 2) |
||||
listPushBack(link, 1) |
||||
|
||||
PrintList(student.ListSort(link)) |
||||
|
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/student/test$ go build |
||||
student@ubuntu:~/student/test$ ./test |
||||
0 -> 1 -> 2 -> 3 -> 4 -> 5 -> <nil> |
||||
student@ubuntu:~/student/test$ |
||||
``` |
@ -0,0 +1,5 @@
|
||||
# onlya |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that displays a 'a' character on the standard output. |
@ -0,0 +1,34 @@
|
||||
# Point |
||||
|
||||
## Instructions |
||||
|
||||
Create a `.go` file and copy the code below into our file |
||||
|
||||
- The main task is to return a working program. |
||||
|
||||
- |
||||
|
||||
```go |
||||
func setPoint(ptr *point) { |
||||
ptr.x = 42 |
||||
ptr.y = 21 |
||||
} |
||||
|
||||
func main() { |
||||
points := &point{} |
||||
|
||||
setPoint(points) |
||||
|
||||
fmt.Printf("x = %d, y = %d",points.x, points.y) |
||||
fmt.Println() |
||||
} |
||||
``` |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
x = 42, y = 21 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,56 @@
|
||||
# Rectangle |
||||
|
||||
## Instructions |
||||
|
||||
Consider that a point is defined by its coordinates and that a rectangle |
||||
is defined by the points of the upper left and lower right corners. |
||||
|
||||
- Define two structures named, `point` and `rectangle`. |
||||
|
||||
- The struct `point` has to have two variables, `x` and `y`, type `int`. |
||||
|
||||
- The struct `rectangle` has to have two variables, `upLeft` and `downRight` type `point`. |
||||
|
||||
- Our main task is to make a program that: |
||||
|
||||
- Given a slice of points of size `n` returns the smallest rectangle that contains all the points in the vector of points. The name of that function is `defineRectangle` |
||||
|
||||
- And calculates and prints the area of that rectangle you define. |
||||
|
||||
## Expected main and function for the program |
||||
|
||||
```go |
||||
func defineRectangle(ptr *point, n int) *rectangle { |
||||
//complete here |
||||
} |
||||
|
||||
func calArea(ptr *rectangle) int { |
||||
//complete here |
||||
} |
||||
|
||||
func main() { |
||||
vPoint := []point{} |
||||
rectangle := &rectangle{} |
||||
n := 7 |
||||
|
||||
for i := 0; i < n; i++ { |
||||
val := point{ |
||||
x: i%2 + 1, |
||||
y: i + 2, |
||||
} |
||||
vPoint = append(vPoint, val) |
||||
} |
||||
rectangle = defineRectangle(vPoint, n) |
||||
fmt.Println("area of the rectangle:", calArea(rectangle)) |
||||
} |
||||
``` |
||||
|
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
area of the rectangle: 6 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,25 @@
|
||||
# rot13 |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that takes a string and displays it, replacing each of its |
||||
letters by the letter 13 spaces ahead in alphabetical order. |
||||
|
||||
- 'z' becomes 'm' and 'Z' becomes 'M'. Case remains unaffected. |
||||
|
||||
- The output will be followed by a newline. |
||||
|
||||
- If the number of arguments is not 1, the program displays a newline. |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "abc" |
||||
nop |
||||
student@ubuntu:~/piscine/test$ ./test "hello there" |
||||
uryyb gurer |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,46 @@
|
||||
# ROT 14 |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `rot14` that returns the string within the parameter but transformed into a rot14 string. |
||||
|
||||
- If you not certain what we are talking about, there is a rot13 already. |
||||
|
||||
## Expected function |
||||
|
||||
```go |
||||
func rot14(str string) string { |
||||
|
||||
} |
||||
``` |
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func main() { |
||||
result := rot14("Hello How are You") |
||||
arrayRune := []rune(result) |
||||
|
||||
for _, s := range arrayRune { |
||||
z01.PrintRune(s) |
||||
} |
||||
z01.PrintRune('\n') |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
Vszzc Vck ofs Mci |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,22 @@
|
||||
# searchreplace |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that takes 3 arguments, the first arguments is a string in which to replace a letter (2nd argument) by another one (3rd argument). |
||||
|
||||
- If the number of arguments is not 3, just display a newline. |
||||
|
||||
- If the second argument is not contained in the first one (the string) then the program simply rewrites the string followed by a newline. |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "hella there" "a" "o" |
||||
hello there |
||||
student@ubuntu:~/piscine/test$ ./test "abcd" "z" "l" |
||||
abcd |
||||
student@ubuntu:~/piscine/test$ ./test "something" "a" "o" "b" "c" |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,71 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `SortedListMerge` that mereges two lists, `l1` and `l2`, but you have to join them in ascending order. |
||||
|
||||
- Tip each list as to be already sorted, and initialized with 0. |
||||
|
||||
- Use pointers when ever you can. |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Nodee struct { |
||||
Data interface{} |
||||
Next *Nodee |
||||
} |
||||
|
||||
func SortedListMerge(l1 *Nodee, l2 *Nodee) *Nodee { |
||||
|
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
func PrintList(l *Nodee) { |
||||
m := l |
||||
for m != nil { |
||||
fmt.Print(m.Data, " -> ") |
||||
m = m.Next |
||||
} |
||||
|
||||
fmt.Print(nil) |
||||
fmt.Println() |
||||
} |
||||
|
||||
func main() { |
||||
link := &Nodee{} |
||||
link2 := &Nodee{} |
||||
|
||||
piscine.ListPushBack(link, "5") |
||||
piscine.ListPushBack(link, "3") |
||||
piscine.ListPushBack(link, "7") |
||||
|
||||
piscine.ListPushBack(link2, "1") |
||||
piscine.ListPushBack(link2, "-2") |
||||
piscine.ListPushBack(link2, "4") |
||||
piscine.ListPushBack(link2, "6") |
||||
|
||||
PrintList(SortedListMerge(link, link2)) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
-2 -> 0 -> 0 -> 1 -> 3 -> 4 -> 5 -> 6 -> 7 -> <nil> |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,87 @@
|
||||
# listpushback |
||||
|
||||
## Instructions |
||||
|
||||
Write a function `SortListInsert` that inserts `Data_ref` in the linked list, but it as to remain sorted in ascending order. |
||||
|
||||
- The list as to be alredy sorted. |
||||
|
||||
- Use pointers when ever you can. |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
type Nodee struct { |
||||
Data int |
||||
Next *Nodee |
||||
} |
||||
|
||||
func SortListInsert(l *Nodee, Data_ref int) *Nodee{ |
||||
|
||||
} |
||||
``` |
||||
|
||||
## Usage |
||||
|
||||
Here is a possible [program](TODO-LINK) to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
piscine ".." |
||||
) |
||||
|
||||
//Prints the list |
||||
func PrintList(l *Nodee) { |
||||
m := l |
||||
for m != nil { |
||||
fmt.Print(m.Data, " -> ") |
||||
m = m.Next |
||||
} |
||||
fmt.Print(nil) |
||||
fmt.Println() |
||||
} |
||||
//insert elements |
||||
func listPushBack(l *Nodee, Data int) { |
||||
n := &Nodee{} |
||||
n.Data = Data |
||||
n.Next = nil |
||||
if l == nil { |
||||
l = n |
||||
return |
||||
} |
||||
iterator := l |
||||
for iterator.Next != nil { |
||||
iterator = iterator.Next |
||||
} |
||||
iterator.Next = n |
||||
} |
||||
|
||||
func main() { |
||||
|
||||
link := &Nodee{} |
||||
|
||||
listPushBack(link, 1) |
||||
listPushBack(link, 4) |
||||
listPushBack(link, 9) |
||||
|
||||
PrintList(link) |
||||
|
||||
link = sortListInsert(link, -2) |
||||
link = sortListInsert(link, 2) |
||||
PrintList(link) |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
-2 -> 0 -> 1 -> 2 -> 4 -> 9 -> <nil> |
||||
lee@lee:~/Documents/work/day11/11-16-sortlistinsert/so |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,24 @@
|
||||
# strlen |
||||
|
||||
## Instructions |
||||
|
||||
Write a function that returns the length of a string. |
||||
|
||||
- `len` is forbidden |
||||
|
||||
## Expected function and structure |
||||
|
||||
```go |
||||
func Strlen(str string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
4 |
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,22 @@
|
||||
# switchcase |
||||
|
||||
## Instructions |
||||
|
||||
Write a program that takes a string and reverses the case of all its letters. |
||||
|
||||
- Other characters remain unchanged. |
||||
|
||||
- You must display the result followed by a '\n'. |
||||
|
||||
- If the number of arguments is not 1, the program displays '\n'. |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "SometHingS iS WronG" |
||||
sOMEThINGs Is wRONg |
||||
student@ubuntu:~/piscine/test$ ./test |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
@ -0,0 +1,25 @@
|
||||
# switchcase |
||||
|
||||
## Instructions |
||||
|
||||
|
||||
Write a program that takes two strings and checks whether it's possible to write the first string with characters from the second string, while respecting the order in which these characters appear in the second string. |
||||
|
||||
- If it's possible, the program displays the string followed by a `\n`, otherwise it simply displays a `\n`. |
||||
|
||||
- If the number of arguments is not 2, the program displays `\n`. |
||||
|
||||
## Expected output |
||||
|
||||
```console |
||||
student@ubuntu:~/piscine/test$ go build |
||||
student@ubuntu:~/piscine/test$ ./test "faya" "fgvvfdxcacpolhyghbreda" |
||||
faya |
||||
student@ubuntu:~/piscine/test$ ./test "faya" "fgvvfdxcacpolhyghbred" |
||||
|
||||
student@ubuntu:~/piscine/test$ ./test "error" |
||||
|
||||
student@ubuntu:~/piscine/test$ ./test |
||||
|
||||
student@ubuntu:~/piscine/test$ |
||||
``` |
Loading…
Reference in new issue