mirror of https://github.com/01-edu/public.git
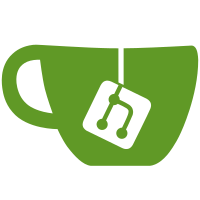
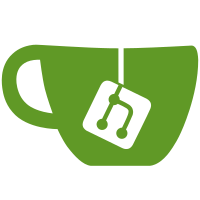
52 changed files with 342 additions and 720 deletions
@ -1,59 +0,0 @@
|
||||
## atoi |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that simulates the behaviour of the `Atoi` function in Go. `Atoi` transforms a number represented as a `string` in a number represented as an `int`. |
||||
|
||||
- `Atoi` returns `0` if the `string` is not considered as a valid number. For this exercise **non-valid `string` chains will be tested**. Some will contain non-digits characters. |
||||
|
||||
- For this exercise the handling of the signs `+` or `-` **does have** to be taken into account. |
||||
|
||||
- This function will **only** have to return the `int`. For this exercise the `error` result of `Atoi` is not required. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Atoi(s string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
fmt.Println(Atoi("12345")) |
||||
fmt.Println(Atoi("0000000012345")) |
||||
fmt.Println(Atoi("012 345")) |
||||
fmt.Println(Atoi("Hello World!")) |
||||
fmt.Println(Atoi("+1234")) |
||||
fmt.Println(Atoi("-1234")) |
||||
fmt.Println(Atoi("++1234")) |
||||
fmt.Println(Atoi("--1234")) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
12345 |
||||
12345 |
||||
0 |
||||
0 |
||||
1234 |
||||
-1234 |
||||
0 |
||||
0 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- [strconv/Atoi](https://golang.org/pkg/strconv/#Atoi) |
@ -0,0 +1,17 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(piscine.Atoi("12345")) |
||||
fmt.Println(piscine.Atoi("0000000012345")) |
||||
fmt.Println(piscine.Atoi("012 345")) |
||||
fmt.Println(piscine.Atoi("Hello World!")) |
||||
fmt.Println(piscine.Atoi("+1234")) |
||||
fmt.Println(piscine.Atoi("-1234")) |
||||
fmt.Println(piscine.Atoi("++1234")) |
||||
fmt.Println(piscine.Atoi("--1234")) |
||||
} |
@ -1,62 +0,0 @@
|
||||
## atoibase |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that takes two arguments: |
||||
|
||||
- `s`: a numeric `string` in a given [base](<https://simple.wikipedia.org/wiki/Base_(mathematics)>). |
||||
- `base`: a `string` representing all the different digits that can represent a numeric value. |
||||
|
||||
And return the integer value of `s` in the given `base`. |
||||
|
||||
If the base is not valid it returns `0`. |
||||
|
||||
Validity rules for a base : |
||||
|
||||
- A base must contain at least 2 characters. |
||||
- Each character of a base must be unique. |
||||
- A base should not contain `+` or `-` characters. |
||||
|
||||
String number must contain only elements that are in base. |
||||
|
||||
Only valid `string` numbers will be tested. |
||||
|
||||
The function **does not have** to manage negative numbers. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func AtoiBase(s string, base string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
fmt.Println(AtoiBase("125", "0123456789")) |
||||
fmt.Println(AtoiBase("1111101", "01")) |
||||
fmt.Println(AtoiBase("7D", "0123456789ABCDEF")) |
||||
fmt.Println(AtoiBase("uoi", "choumi")) |
||||
fmt.Println(AtoiBase("bbbbbab", "-ab")) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
125 |
||||
125 |
||||
125 |
||||
125 |
||||
0 |
||||
$ |
||||
``` |
@ -0,0 +1,14 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(piscine.AtoiBase("125", "0123456789")) |
||||
fmt.Println(piscine.AtoiBase("1111101", "01")) |
||||
fmt.Println(piscine.AtoiBase("7D", "0123456789ABCDEF")) |
||||
fmt.Println(piscine.AtoiBase("uoi", "choumi")) |
||||
fmt.Println(piscine.AtoiBase("bbbbbab", "-ab")) |
||||
} |
@ -0,0 +1,11 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(piscine.BeZero([]int{1, 2, 3, 4, 5, 6})) |
||||
fmt.Println(piscine.BeZero([]int{})) |
||||
} |
@ -0,0 +1,14 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(piscine.BinaryCheck(5)) |
||||
fmt.Println(piscine.BinaryCheck(0)) |
||||
fmt.Println(piscine.BinaryCheck(8)) |
||||
fmt.Println(piscine.BinaryCheck(-9)) |
||||
fmt.Println(piscine.BinaryCheck(-4)) |
||||
} |
@ -1,43 +0,0 @@
|
||||
## compare |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that behaves like the `Compare` function. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Compare(a, b string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
fmt.Println(Compare("Hello!", "Hello!")) |
||||
fmt.Println(Compare("Salut!", "lut!")) |
||||
fmt.Println(Compare("Ola!", "Ol")) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
0 |
||||
-1 |
||||
1 |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- [strings/Compare](https://golang.org/pkg/strings/#Compare) |
@ -1,9 +0,0 @@
|
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
fmt.Println(Compare("Hello!", "Hello!")) |
||||
fmt.Println(Compare("Salut!", "lut!")) |
||||
fmt.Println(Compare("Ola!", "Ol")) |
||||
} |
@ -0,0 +1,12 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(piscine.ConcatSlice([]int{1, 2, 3}, []int{4, 5, 6})) |
||||
fmt.Println(piscine.ConcatSlice([]int{}, []int{4, 5, 6, 7, 8, 9})) |
||||
fmt.Println(piscine.ConcatSlice([]int{1, 2, 3}, []int{})) |
||||
} |
@ -0,0 +1,12 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Print(piscine.FifthAndSkip("abcdefghijklmnopqrstuwxyz")) |
||||
fmt.Print(piscine.FifthAndSkip("This is a short sentence")) |
||||
fmt.Print(piscine.FifthAndSkip("1234")) |
||||
} |
@ -1,44 +0,0 @@
|
||||
## firstrune |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that returns the first `rune` of a `string`. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func FirstRune(s string) rune { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func main() { |
||||
z01.PrintRune(FirstRune("Hello!")) |
||||
z01.PrintRune(FirstRune("Salut!")) |
||||
z01.PrintRune(FirstRune("Ola!")) |
||||
z01.PrintRune('\n') |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
HSO |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- [rune-literals](https://golang.org/ref/spec#Rune_literals) |
@ -0,0 +1,14 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
|
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
z01.PrintRune(piscine.FirstRune("Hello!")) |
||||
z01.PrintRune(piscine.FirstRune("Salut!")) |
||||
z01.PrintRune(piscine.FirstRune("Ola!")) |
||||
z01.PrintRune('\n') |
||||
} |
@ -0,0 +1,12 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(piscine.FishAndChips(4)) |
||||
fmt.Println(piscine.FishAndChips(9)) |
||||
fmt.Println(piscine.FishAndChips(6)) |
||||
} |
@ -0,0 +1,12 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Print(piscine.FromTo(1, 10)) |
||||
fmt.Print(piscine.FromTo(10, 1)) |
||||
fmt.Print(piscine.FromTo(10, 10)) |
||||
fmt.Print(piscine.FromTo(100, 10)) |
||||
} |
@ -1,44 +0,0 @@
|
||||
## lastrune |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that returns the last `rune` of a `string`. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func LastRune(s string) rune { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func main() { |
||||
z01.PrintRune(LastRune("Hello!")) |
||||
z01.PrintRune(LastRune("Salut!")) |
||||
z01.PrintRune(LastRune("Ola!")) |
||||
z01.PrintRune('\n') |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
!!! |
||||
$ |
||||
``` |
||||
|
||||
### Notions |
||||
|
||||
- [rune-literals](https://golang.org/ref/spec#Rune_literals) |
@ -0,0 +1,14 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
|
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
z01.PrintRune(piscine.LastRune("Hello!")) |
||||
z01.PrintRune(piscine.LastRune("Salut!")) |
||||
z01.PrintRune(piscine.LastRune("Ola!")) |
||||
z01.PrintRune('\n') |
||||
} |
@ -1,102 +0,0 @@
|
||||
## listremoveif |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `ListRemoveIf` that removes all elements that are equal to the `data_ref` in the argument of the function. |
||||
|
||||
### Expected function and structure |
||||
|
||||
```go |
||||
type NodeL struct { |
||||
Data interface{} |
||||
Next *NodeL |
||||
} |
||||
|
||||
type List struct { |
||||
Head *NodeL |
||||
Tail *NodeL |
||||
} |
||||
|
||||
func ListRemoveIf(l *List, data_ref interface{}) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func PrintList(l *List) { |
||||
it := l.Head |
||||
for it != nil { |
||||
fmt.Print(it.Data, " -> ") |
||||
it = it.Next |
||||
} |
||||
|
||||
fmt.Print(nil, "\n") |
||||
} |
||||
|
||||
func main() { |
||||
link := &List{} |
||||
link2 := &List{} |
||||
|
||||
fmt.Println("----normal state----") |
||||
ListPushBack(link2, 1) |
||||
PrintList(link2) |
||||
ListRemoveIf(link2, 1) |
||||
fmt.Println("------answer-----") |
||||
PrintList(link2) |
||||
fmt.Println() |
||||
|
||||
fmt.Println("----normal state----") |
||||
ListPushBack(link, 1) |
||||
ListPushBack(link, "Hello") |
||||
ListPushBack(link, 1) |
||||
ListPushBack(link, "There") |
||||
ListPushBack(link, 1) |
||||
ListPushBack(link, 1) |
||||
ListPushBack(link, "How") |
||||
ListPushBack(link, 1) |
||||
ListPushBack(link, "are") |
||||
ListPushBack(link, "you") |
||||
ListPushBack(link, 1) |
||||
PrintList(link) |
||||
|
||||
ListRemoveIf(link, 1) |
||||
fmt.Println("------answer-----") |
||||
PrintList(link) |
||||
} |
||||
|
||||
func ListPushBack(l *List, data interface{}) { |
||||
n := &NodeL{Data: data} |
||||
if l.Head == nil { |
||||
l.Head = n |
||||
l.Tail = n |
||||
} else { |
||||
l.Tail.Next = n |
||||
l.Tail = n |
||||
} |
||||
} |
||||
|
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
----normal state---- |
||||
1 -> <nil> |
||||
------answer----- |
||||
<nil> |
||||
|
||||
----normal state---- |
||||
1 -> Hello -> 1 -> There -> 1 -> 1 -> How -> 1 -> are -> you -> 1 -> <nil> |
||||
------answer----- |
||||
Hello -> There -> How -> are -> you -> <nil> |
||||
$ |
||||
``` |
@ -1,55 +0,0 @@
|
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func PrintList(l *List) { |
||||
it := l.Head |
||||
for it != nil { |
||||
fmt.Print(it.Data, " -> ") |
||||
it = it.Next |
||||
} |
||||
|
||||
fmt.Print(nil, "\n") |
||||
} |
||||
|
||||
func main() { |
||||
link := &List{} |
||||
link2 := &List{} |
||||
|
||||
fmt.Println("----normal state----") |
||||
ListPushBack(link2, 1) |
||||
PrintList(link2) |
||||
ListRemoveIf(link2, 1) |
||||
fmt.Println("------answer-----") |
||||
PrintList(link2) |
||||
fmt.Println() |
||||
|
||||
fmt.Println("----normal state----") |
||||
ListPushBack(link, 1) |
||||
ListPushBack(link, "Hello") |
||||
ListPushBack(link, 1) |
||||
ListPushBack(link, "There") |
||||
ListPushBack(link, 1) |
||||
ListPushBack(link, 1) |
||||
ListPushBack(link, "How") |
||||
ListPushBack(link, 1) |
||||
ListPushBack(link, "are") |
||||
ListPushBack(link, "you") |
||||
ListPushBack(link, 1) |
||||
PrintList(link) |
||||
|
||||
ListRemoveIf(link, 1) |
||||
fmt.Println("------answer-----") |
||||
PrintList(link) |
||||
} |
||||
|
||||
func ListPushBack(l *List, data interface{}) { |
||||
n := &NodeL{Data: data} |
||||
if l.Head == nil { |
||||
l.Head = n |
||||
l.Tail = n |
||||
} else { |
||||
l.Tail.Next = n |
||||
l.Tail = n |
||||
} |
||||
} |
@ -1,37 +0,0 @@
|
||||
## max |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `Max` that will return the maximum value in a slice of integers. If the slice is empty it will return 0. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Max(a []int) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
a := []int{23, 123, 1, 11, 55, 93} |
||||
max := Max(a) |
||||
fmt.Println(max) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
123 |
||||
$ |
||||
``` |
@ -0,0 +1,12 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
a := []int{23, 123, 1, 11, 55, 93} |
||||
max := piscine.Max(a) |
||||
fmt.Println(max) |
||||
} |
@ -1,43 +0,0 @@
|
||||
## printcomb |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that prints, in ascending order and on a single line: all **unique** combinations of three different digits so that, the first digit is lower than the second, and the second is lower than the third. |
||||
|
||||
These combinations are separated by a comma and a space. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func PrintComb() { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
func main() { |
||||
PrintComb() |
||||
} |
||||
``` |
||||
|
||||
This is the incomplete output : |
||||
|
||||
```console |
||||
$ go run . | cat -e |
||||
012, 013, 014, 015, 016, 017, 018, 019, 023, ..., 689, 789$ |
||||
$ |
||||
``` |
||||
|
||||
- `000` or `999` are not valid combinations because the digits are not different. |
||||
|
||||
- `987` should not be shown because the first digit is not less than the second. |
||||
|
||||
### Notions |
||||
|
||||
- [01-edu/z01](https://github.com/01-edu/z01) |
@ -0,0 +1,7 @@
|
||||
package main |
||||
|
||||
import "piscine" |
||||
|
||||
func main() { |
||||
piscine.PrintComb() |
||||
} |
@ -0,0 +1,7 @@
|
||||
package main |
||||
|
||||
import "piscine" |
||||
|
||||
func main() { |
||||
piscine.PrintMemory([10]byte{'h', 'e', 'l', 'l', 'o', 16, 21, '*'}) |
||||
} |
@ -0,0 +1,13 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(piscine.RetainFirstHalf("This is the 1st halfThis is the 2nd half")) |
||||
fmt.Println(piscine.RetainFirstHalf("A")) |
||||
fmt.Println(piscine.RetainFirstHalf("")) |
||||
fmt.Println(piscine.RetainFirstHalf("Hello World")) |
||||
} |
@ -0,0 +1,13 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(piscine.RevConcatAlternate([]int{1, 2, 3}, []int{4, 5, 6})) |
||||
fmt.Println(piscine.RevConcatAlternate([]int{1, 2, 3}, []int{4, 5, 6, 7, 8, 9})) |
||||
fmt.Println(piscine.RevConcatAlternate([]int{1, 2, 3, 9, 8}, []int{4, 5})) |
||||
fmt.Println(piscine.RevConcatAlternate([]int{1, 2, 3}, []int{})) |
||||
} |
@ -1,45 +0,0 @@
|
||||
## rot14 |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `rot14` that returns the `string` within the parameter transformed into a `rot14 string`. |
||||
Each letter will be replaced by the letter 14 spots ahead in the alphabetical order. |
||||
|
||||
- 'z' becomes 'n' and 'Z' becomes 'N'. The case of the letter stays the same. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Rot14(s string) string { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import ( |
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func main() { |
||||
result := Rot14("Hello! How are You?") |
||||
|
||||
for _, r := range result { |
||||
z01.PrintRune(r) |
||||
} |
||||
z01.PrintRune('\n') |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
Vszzc! Vck ofs Mci? |
||||
$ |
||||
``` |
@ -0,0 +1,16 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"piscine" |
||||
|
||||
"github.com/01-edu/z01" |
||||
) |
||||
|
||||
func main() { |
||||
result := piscine.Rot14("Hello! How are You?") |
||||
|
||||
for _, r := range result { |
||||
z01.PrintRune(r) |
||||
} |
||||
z01.PrintRune('\n') |
||||
} |
@ -0,0 +1,15 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Println(piscine.SaveAndMiss("123456789", 3)) |
||||
fmt.Println(piscine.SaveAndMiss("abcdefghijklmnopqrstuvwyz", 3)) |
||||
fmt.Println(piscine.SaveAndMiss("", 3)) |
||||
fmt.Println(piscine.SaveAndMiss("hello you all ! ", 0)) |
||||
fmt.Println(piscine.SaveAndMiss("what is your name?", 0)) |
||||
fmt.Println(piscine.SaveAndMiss("go Exercise Save and Miss", -5)) |
||||
} |
@ -0,0 +1,15 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
a := []string{"coding", "algorithm", "ascii", "package", "golang"} |
||||
fmt.Printf("%#v\n", piscine.Slice(a, 1)) |
||||
fmt.Printf("%#v\n", piscine.Slice(a, 2, 4)) |
||||
fmt.Printf("%#v\n", piscine.Slice(a, -3)) |
||||
fmt.Printf("%#v\n", piscine.Slice(a, -2, -1)) |
||||
fmt.Printf("%#v\n", piscine.Slice(a, 2, 0)) |
||||
} |
@ -1,38 +0,0 @@
|
||||
## sortwordarr |
||||
|
||||
### Instructions |
||||
|
||||
Write a function `SortWordArr` that sorts by `ascii` (in ascending order) a `string` slice. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func SortWordArr(a []string) { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
result := []string{"a", "A", "1", "b", "B", "2", "c", "C", "3"} |
||||
SortWordArr(result) |
||||
|
||||
fmt.Println(result) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
[1 2 3 A B C a b c] |
||||
$ |
||||
``` |
@ -1,9 +0,0 @@
|
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
result := []string{"a", "A", "1", "b", "B", "2", "c", "C", "3"} |
||||
SortWordArr(result) |
||||
fmt.Println(result) |
||||
} |
@ -1,36 +0,0 @@
|
||||
## split |
||||
|
||||
### Instructions |
||||
|
||||
Write a function that receives a string and a separator and returns a `slice of strings` that results of splitting the string `s` by the separator `sep`. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func Split(s, sep string) []string { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
s := "HelloHAhowHAareHAyou?" |
||||
fmt.Printf("%#v\n", Split(s, "HA")) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
[]string{"Hello", "how", "are", "you?"} |
||||
$ |
||||
``` |
@ -0,0 +1,11 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
s := "HelloHAhowHAareHAyou?" |
||||
fmt.Printf("%#v\n", piscine.Split(s, "HA")) |
||||
} |
@ -1,36 +0,0 @@
|
||||
## strlen |
||||
|
||||
### Instructions |
||||
|
||||
- Write a function that counts the `runes` of a `string` and that returns that count. |
||||
|
||||
### Expected function |
||||
|
||||
```go |
||||
func StrLen(s string) int { |
||||
|
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible program to test your function : |
||||
|
||||
```go |
||||
package main |
||||
|
||||
import "fmt" |
||||
|
||||
func main() { |
||||
l := StrLen("Hello World!") |
||||
fmt.Println(l) |
||||
} |
||||
``` |
||||
|
||||
And its output : |
||||
|
||||
```console |
||||
$ go run . |
||||
12 |
||||
$ |
||||
``` |
@ -0,0 +1,11 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
l := piscine.StrLen("Hello World!") |
||||
fmt.Println(l) |
||||
} |
@ -0,0 +1,13 @@
|
||||
package main |
||||
|
||||
import ( |
||||
"fmt" |
||||
"piscine" |
||||
) |
||||
|
||||
func main() { |
||||
fmt.Print(piscine.WordFlip("First second last")) |
||||
fmt.Print(piscine.WordFlip("")) |
||||
fmt.Print(piscine.WordFlip(" ")) |
||||
fmt.Print(piscine.WordFlip(" hello all of you! ")) |
||||
} |
Loading…
Reference in new issue