mirror of https://github.com/01-edu/public.git
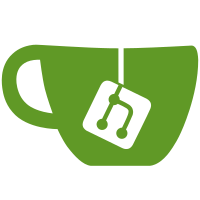
64 changed files with 218 additions and 218 deletions
@ -1,5 +1,5 @@
|
||||
# onlya |
||||
## onlya |
||||
|
||||
## Instructions |
||||
### Instructions |
||||
|
||||
Write a program that displays a 'a' character on the standard output. |
@ -1,4 +1,4 @@
|
||||
# displayalpham |
||||
## Instructions |
||||
## displayalpham |
||||
### Instructions |
||||
|
||||
Write a program that displays a 'z' character on the standard output. |
Loading…
Reference in new issue