mirror of https://github.com/01-edu/public.git
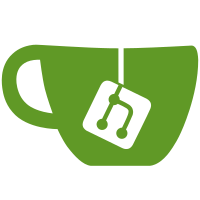
2 changed files with 55 additions and 46 deletions
@ -1,46 +0,0 @@
|
||||
## DoOperation |
||||
|
||||
### Instructions |
||||
|
||||
In a file named `DoOperation.java` write a function `operate` that returns the result of the given arithmetic operation specified in the parameters. The arguments should be passed in the following order: |
||||
|
||||
- The first argument is the left operand |
||||
- The second argument is the operation sign |
||||
- The third argument is the right operand |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class DoOperation { |
||||
public static String operate(String[] args) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible `ExerciseRunner.java` to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(DoOperation.operate(new String[]{"1","+","2"})); |
||||
System.out.println(DoOperation.operate(new String[]{"1","-","1"})); |
||||
System.out.println(DoOperation.operate(new String[]{"1","%","0"})); |
||||
System.out.println(DoOperation.operate(args)); |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
3 |
||||
0 |
||||
Error |
||||
it depend on the input. |
||||
$ |
||||
``` |
@ -0,0 +1,55 @@
|
||||
## UnitConverter |
||||
|
||||
### Instructions |
||||
|
||||
In a file named `UnitConverter.java`, write a function `convert` that returns the result of a unit conversion specified in the parameters. The function should handle different types of unit conversions based on the input parameters which specify the unit from, the unit to, and the value to be converted. |
||||
|
||||
##### Request conversions |
||||
|
||||
- Fahrenheit to Celsius: (F - 32) \* 5/9 |
||||
- Celsius to Fahrenheit: C \* 9/5 + 32 |
||||
- Kilometers to Miles: K \* 0.621371 |
||||
- Miles to Kilometers: M \* 1.60934 |
||||
|
||||
### Expected Functions |
||||
|
||||
```java |
||||
public class UnitConverter { |
||||
public static String convert(String[] args) { |
||||
// your code here |
||||
} |
||||
} |
||||
``` |
||||
|
||||
### Usage |
||||
|
||||
Here is a possible `ExerciseRunner.java` to test your function : |
||||
|
||||
```java |
||||
public class ExerciseRunner { |
||||
public static void main(String[] args) { |
||||
System.out.println(UnitConverter.convert(new String[]{"celsius", "fahrenheit", "100"})); |
||||
System.out.println(UnitConverter.convert(new String[]{"fahrenheit", "celsius", "212"})); |
||||
System.out.println(UnitConverter.convert(new String[]{"kilometers", "miles", "5"})); |
||||
System.out.println(UnitConverter.convert(new String[]{"pounds", "kilograms", "10"})); |
||||
if (args.length == 3) { |
||||
System.out.println(UnitConverter.convert(args)); |
||||
} else { |
||||
System.out.println("Usage: java ExerciseRunner <fromUnit> <toUnit> <value>"); |
||||
} |
||||
} |
||||
} |
||||
``` |
||||
|
||||
and its output : |
||||
|
||||
```shell |
||||
$ javac *.java -d build |
||||
$ java -cp build ExerciseRunner |
||||
212.00 |
||||
100.00 |
||||
3.11 |
||||
4.54 |
||||
it depends on the input. |
||||
$ |
||||
``` |
Loading…
Reference in new issue